PyJive workshop: FrameModel#
import sys
import os
import matplotlib.pyplot as plt
pyjivepath = '../../../pyjive/'
sys.path.append(pyjivepath)
if not os.path.isfile(pyjivepath + 'utils/proputils.py'):
print('\n\n**pyjive cannot be found, adapt "pyjivepath" above or move notebook to appropriate folder**\n\n')
raise Exception('pyjive not found')
from utils import proputils as pu
import main
from names import GlobNames as gn
import contextlib
from urllib.request import urlretrieve
def findfile(fname):
url = "https://gitlab.tudelft.nl/cm/public/drive/-/raw/main/structural/" + fname + "?inline=false"
if not os.path.isfile(fname):
print(f"Downloading {fname}...")
urlretrieve(url, fname)
findfile("vierendeel.geom")
findfile("vierendeel.pro")
findfile("frame.geom")
findfile("frame.pro")
Task 1: Run predefined problems
Familiarize yourself with the FrameModel
and the FrameViewModule
with the two problems for which input files are provided.
Simple frame analysis#
We first analyze a simple frame structure with a point load. Two input files are used:
frame.pro
: The usual input file for pyjive specifying all problem settingsframe.geo
: Instead of a mesh file, a higher level geometry file is used for frame structures. In this file the frame geometry is defined, as well as the number of elements per structural member from which the finite element mesh is created by theInitModule
.
plt.close('all')
props = pu.parse_file('frame.pro')
globdat = main.jive(props)
Vierendeel beam#
Next, we perform analysis on a vierendeel beam
plt.close('all')
props = pu.parse_file('vierendeel.pro')
globdat = main.jive(props)
To inspect some more results, we can create a new FrameViewModule
with properties that are defined in the notebook rather than in the .pro
file. Below we will plot normal force and moment lines in the undeformed mesh.
fv = globdat[gn.MODULEFACTORY].get_module('FrameView','fv')
props['fv'] = {}
props['fv']['plotStress'] = 'N'
props['fv']['deform'] = 0
props['fv']['interactive'] = 'False'
props['fv']['step0'] = 0
fv.init(props, globdat)
status = fv.run(globdat)
fv.shutdown(globdat)
props['fv']['plotStress'] = 'M'
fv.init(props, globdat)
status = fv.run(globdat)
fv.shutdown(globdat)
House-shaped frame#
Task 2: Set up your own problem
Next, define a set of input files (.pro
and .geom
) to perform linear analysis of the frame given below. Not all parameters in the figure are relevant for the linear problem. This case will be revisited in other exercises.
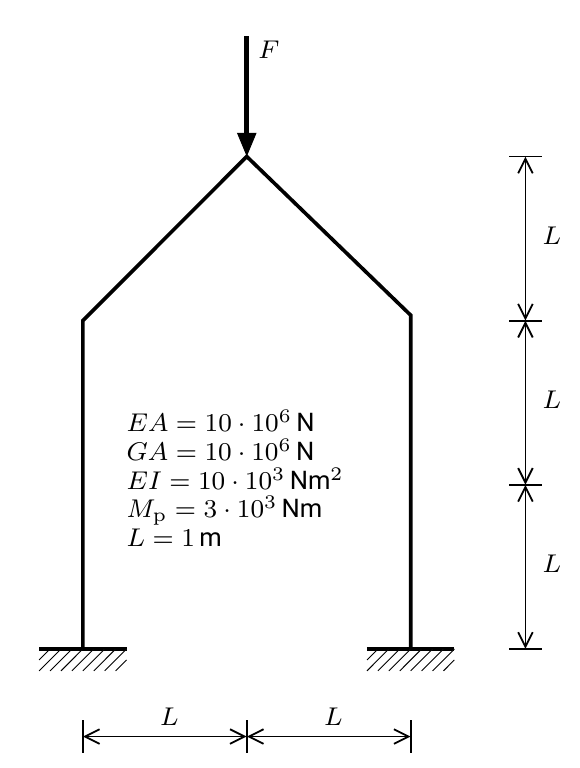